easypoi
简介:
java对excel文件操作的工具类,使用简单,只需简单几个注解即可完成excel的简单导入导出,也可以基于map灵活定义的表头字段(适用于列数不定,动态生成,下面介绍)
使用
maven依赖
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| <dependency> <groupId>cn.afterturn</groupId> <artifactId>easypoi-base</artifactId> <version>4.1.0</version> </dependency> <dependency> <groupId>cn.afterturn</groupId> <artifactId>easypoi-web</artifactId> <version>4.1.0</version> </dependency> <dependency> <groupId>cn.afterturn</groupId> <artifactId>easypoi-annotation</artifactId> <version>4.1.0</version> </dependency>
<dependency> <groupId>cn.afterturn</groupId> <artifactId>easypoi-spring-boot-starter</artifactId> <version>3.3.0</version> </dependency>
|
用于生成excel文件所需数据
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66
|
public static Map<String, Object> transformToExcelMap(Map<String, Object> params) { List<ExcelExportEntity> colList = new ArrayList<>();
colList.add(new ExcelExportEntity("班级", "className")); colList.add(new ExcelExportEntity("学号", "username")); colList.add(new ExcelExportEntity("姓名", "name")); colList.add(new ExcelExportEntity("性别", "sex"));
List<Map<String, Object>> list = new ArrayList<Map<String, Object>>(); List<ExamCorrectDto> examCorrectDtos = (List<ExamCorrectDto>) params.get("data"); examCorrectDtos.forEach(examCorrectDto -> { Map<String, Object> valMap = new HashMap<>(); valMap.put("className", examCorrectDto.getClassName()); valMap.put("username", examCorrectDto.getUsername()); valMap.put("name", examCorrectDto.getName()); list.add(valMap); }); String title = "测验名:" + params.get("examName") + "; 课程:" + params.get("courseName") + "; 日期:" + simpleDateFormat.format(params.get("startTime")) + "; 时长:" + params.get("durationTime") + "; 总题数:" + params.get("questionNum") + "; 总分:" + params.get("totalScore"); ExportParams exportParams = new ExportParams(title, params.get("examName").toString(), ExcelType.XSSF); exportParams.setTitleHeight((short) 15); exportParams.setStyle(ExcelTitleStyleConfig.class);
Map<String, Object> res = new HashMap<>();
res.put(NormalExcelConstants.CLASS, ExcelExportEntity.class); res.put(NormalExcelConstants.DATA_LIST, list); res.put(NormalExcelConstants.PARAMS, exportParams); res.put(NormalExcelConstants.MAP_LIST, colList); return res; }
|
自定义样式
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
|
public class ExcelTitleStyleConfig extends ExcelExportStylerDefaultImpl { public ExcelTitleStyleConfig(Workbook workbook) { super(workbook); }
@Override public CellStyle getHeaderStyle(short color) { CellStyle headStyle = super.getHeaderStyle(color); headStyle.setWrapText(true); headStyle.setFillForegroundColor(IndexedColors.YELLOW.getIndex()); headStyle.setFillPattern(FillPatternType.SOLID_FOREGROUND); return headStyle; } }
|
导出文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
|
public static void exportToExcel(List<Map<String, Object>> paramList) { Workbook workbook = new XSSFWorkbook(); ExcelExportService server = new ExcelExportService(); List<Map<String, Object>> sheetList = new ArrayList(paramList.size()); paramList.forEach(param -> sheetList.add(transformToExcelMap(param))); sheetList.forEach(sheet -> { server.createSheetForMap( workbook, (ExportParams) sheet.get(NormalExcelConstants.PARAMS), (List<ExcelExportEntity>) sheet.get(NormalExcelConstants.MAP_LIST), (List<Map<String, Object>>) sheet.get(NormalExcelConstants.DATA_LIST)); }); FileOutputStream fos = null; try { fos = new FileOutputStream("文件名.xlsx"); workbook.write(fos); } catch (IOException e) { e.printStackTrace(); } finally { if (fos != null) { try { fos.close(); } catch (IOException e) { e.printStackTrace(); } } try { if (workbook != null) { workbook.close(); } } catch (IOException e) { e.printStackTrace(); } } }
|
效果展示
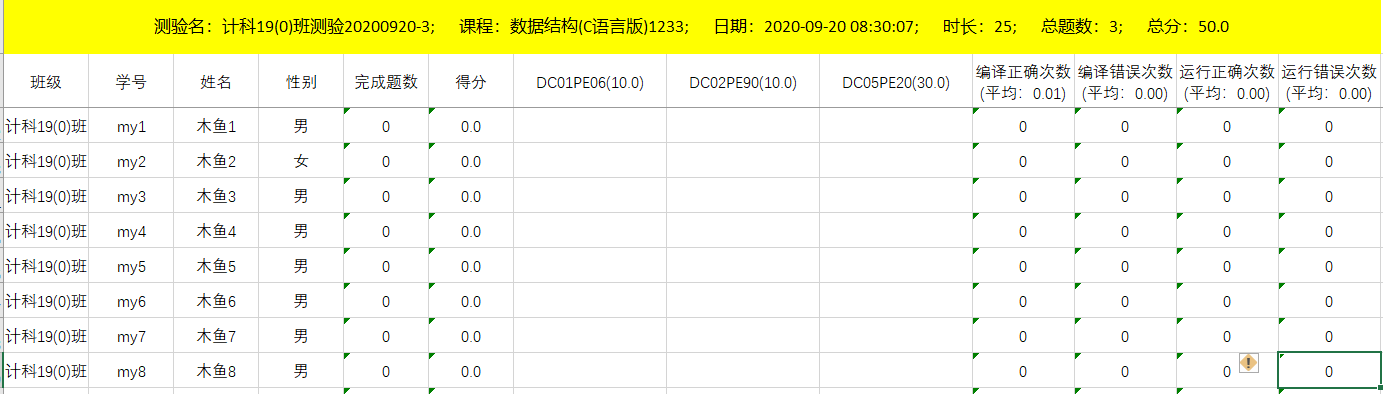
注解形式直接看官方文档即可
参考文档1
参考文档2
注:也可使用easyexcel (阿里巴巴开源的一个excel处理框架,以使用简单、节省内存著称)。
END